Passing ARRAYS to a function
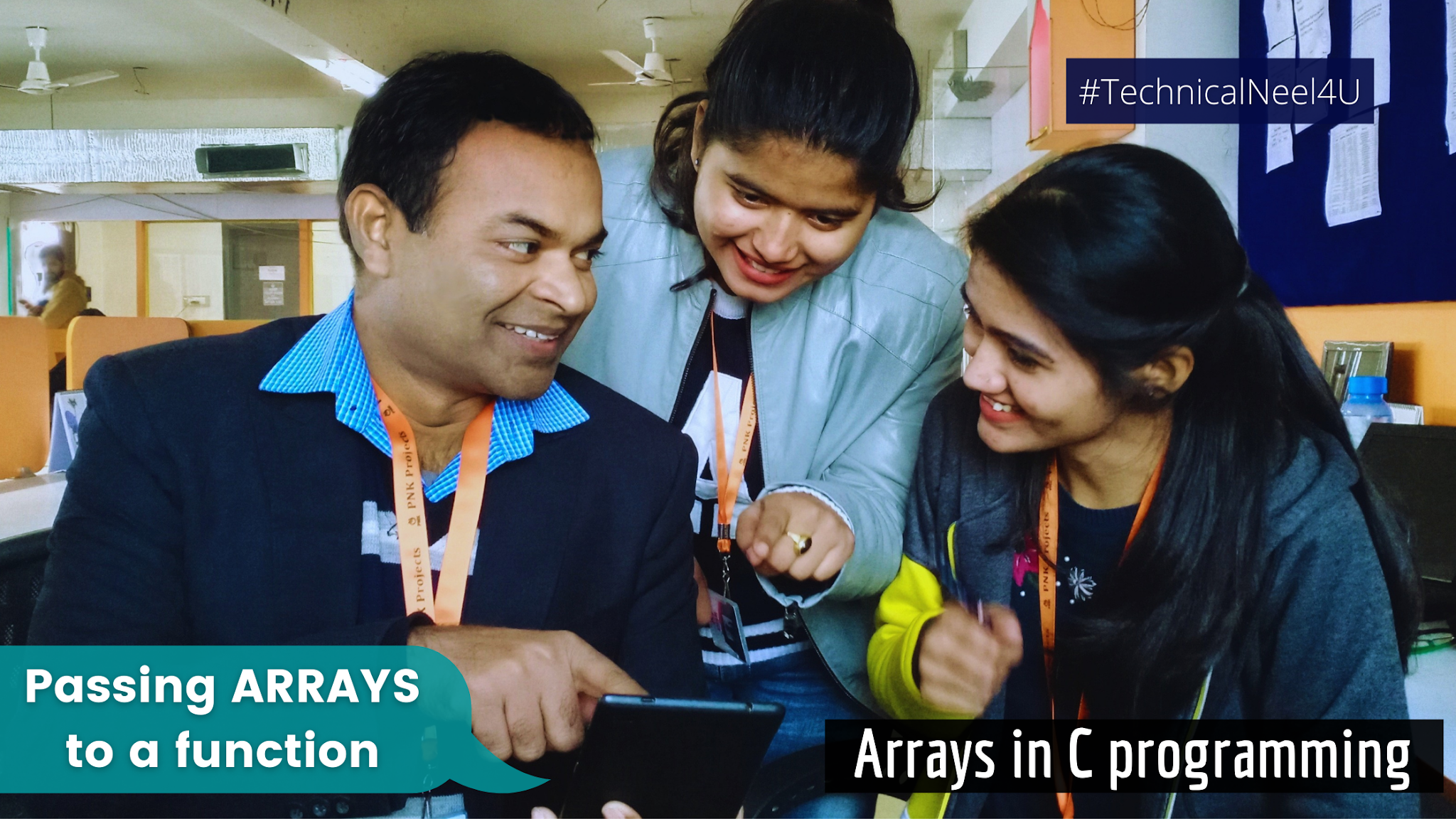
Passing arrays
An array name can be used as an argument to a function, thus permitting the entire array to be passed to the function.
To pass an array to a function, the array name must appear, without brackets or subscripts, as an actual argument within the function call. The corresponding formal argument is written in the same manner, though it must be declared as an array within the formal argument declarations.
when declaring a one-dimensional array as a formal argument, the array name is written with a pair of empty square brackets. The size of the array is not specified within the formal argument declaration.
Example:
void main()
{ int number; /* Variable Declaration */
float avg; /* Variable Declaration */
float table[20];/*Array Definition */
float average(); /* Function Declaration */
avg = average (number, tale);
float average(i, j); /* Function Declaration */
int i; /* formal argument Declaration */
int j[ ]; /* formal argument(array) Declaration */
{ ---
---
}
The function average is called from main, this function call contains two actual arguments - the integer variable number; and the one-dimensional floating-point array table (which appears as an ordinary variable within the function call).
The function definition contains two formal arguments - i & j. The formal argument declarations establish i as an integer variable and j as a one-dimensional, floating-point array.
There is a correspondence between the actual argument number and the formal argument i. Similarly, there is correspondence between the actual argument table and the formal argument j.
Program-1
#include <stdio.h>
#define MAXSIZE 10
/* Sorting in ascending order, a one-dimension integer array. Using Functions */
void main()
{ int array[MAXSIZE];
int i, j;
read(array);
sort(array);
print(array);
}
read(array)
int array[ ];
{ int i;
printf("\nEnter 10 Integers To Be sorted\n");
for(i-0; i<10; i++)
{ printf("Array[%d] = ",i+1);
scanf("% d", &array[i]);
}
return
}
sort(array)
int array[ ];
{ int i, j, temp;
for(i=0; i<9; i++)
for(j=i+1; j<10; j++)
if(array[i] < array[j])
{ temp = array[i];
array[i] = array[j];
array[j] = temp;
}
return;
}
print(array)
int array[ ];
{ int i;
printf("\nArray Sorted in Descending Order ");
for(i=0; i<10; i++)
printf("\nArray[%d] = %d", i+1, array[i]);
return;
}
If any of the arguments are arrays, an empty pair of square brackets must follow the data type of each array argument, thus indicating that the argument is an array.
If the first line of a function definition includes the formal argument declarations, each array name appearing as a formal argument must be followed by an empty pair of square brackets.
Arguments are passed to a function by value when the arguments are ordinary variables. When an array is passed to a function, the values of the array elements are not passed to the function. Rather, the array name is interpreted as the address of the first array element. Arguments passed in this manner are said to be passed by reference rather than by value.
When a reference is made to an array element within the function, the value of the elements subscript is added to the value of the pointer to indicate the address of the specified array element.
If an array element is changed within the function, the changes will be recognized in the calling portion of the program.
Program-2
#include <stdio.h>
/* Effects of changes, while passing an array to a function */
void main()
{ int count, a[3];
void modify(int a[]);
printf("\n This is From Main Program\n");
for(count=0; count<=2; ++count)
{ a[count] = count +1;
printf("a[%d] = %d\n", count, a[count]);
}
modify(a);
printf("\n This Is Again From Main Program\n");
{ a[count] = count +1;
printf("a[%d] = %d\n", count, a[count]);
}
}
void modify(int a[ ])
{ int count;
printf("\n This Is From Called Function\n");
for(count=0; count<=2; ++count){ a[count]= 9;
printf("a[%d] = %d\n", count, a[count]);
}
return;
Read Also:
Multidimensional Arrays in C Programming
Exercise regularly. Eat a nutritious diet. Don't smoke - WHO (Covid-19).
- Learn how to write the C program step-by-step | Lesson -1
- Learn how to write the C program step-by-step | Lesson -2
- Learn how to write the C program step-by-step | Lesson -3
- Learn how to write the C program step-by-step | Lesson -4
- Learn how to write the C program step-by-step | Lesson -5
- Learn how to write the C program step-by-step | Lesson -6
- Learn how to write the C program step-by-step | Lesson -7
Let's see also...
- Revolution in Information Technology
- Important Terminology of Computer Science
- Most useful website list for job seekers
- Do you know how to delete Facebook account
- What is TCP/IP Reference Model
No comments:
For More Details Subscribe & Comment..!